Create React Native apps UI easily and fastly ⚡️
Ficus UI is a simple, modular, and accessible UI library for React Native, forked from Magnus UI and inspired by Chakra UI
<VStack
p={10}
borderWidth={1}
borderColor="gray.200"
borderRadius="lg"
spacing="md"
bg="white"
>
<Image
borderRadius="md"
source={{ uri: 'https://bit.ly/4dR2Hgy' }}
w="100%"
h={200}
alt="House image"
/>
<HStack alignItems="center" mt={2} spacing="sm">
<Badge colorScheme="pink" fontSize="md">Plus</Badge>
<Text
textTransform="uppercase"
fontSize="sm"
fontWeight="bold"
color="pink.800"
>
Cape Town
</Text>
</HStack>
<Text fontSize="xl" fontWeight="bold" lineHeight="short">
Modern, Chic Penthouse with Pool and Golf park
</Text>
<Text fontSize="lg">200$/night</Text>
<Box flexDirection="row" alignItems="center">
<Text fontSize="sm">⭐️</Text>
<Text ml={5} fontSize="sm" fontWeight="bold">
4.91 (290)
</Text>
</Box>
</VStack>
Plus
Cape Town
Modern, Chic Penthouse with Pool and Golf park
200$/night
⭐️
4.91 (290)
Style props
React Native Ficus UI allows you to pass all the style properties as component props. You don't need to create huge StyleSheet objectsTheme support
You can edit the components sizes, colors, borders, ... with your own theme.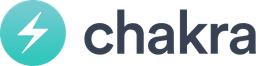
Chakra UI for React Native
React Native Ficus UI provides the support of style props on React Native components and some components implemented as from Chakra UI (with same API).Responsive has never been easier 🤩
<Box w="100%">
<Box
flexDirection="row"
justify={['flex-start', 'center']}
my="lg"
>
<Box
flexDirection={['column', 'row']}
w={['100%', undefined]}
>
<Input
placeholder="Search location"
p={10}
w={['100%', 300]}
borderWidth={2}
focusBorderColor="teal.500"
/>
<Button
colorScheme="teal"
full={[true, false]}
mt={[5, 0]}
ml={[0, 5]}
>
Search
</Button>
</Box>
</Box>
<Box mt="lg">
<ItemCard content={item} w={['100%', '50%']} />
</Box>
</Box>
Customize the theme as you want 🎨
Example of text with font size xs
Example of text with font size sm
Example of text with font size md
Example of text with font size lg
Example of text with font size xl
Example of text with font size 2xl
Example of text with font size 3xl
Example of text with font size 4xl
export const Theme = () => {
const theme = {
colors: {
brand: {
50: '#ddfff3',
100: '#b5f6e0',
200: '#8cf0cd',
300: '#60e8ba',
400: '#36e2a6',
500: '#1dc98d',
600: '#119c6d',
700: '#046f4d',
800: '#00442e',
900: '#00180e',
},
violet: {
50: '#f0eaff',
100: '#d1c1f4',
200: '#b199e7',
300: '#9171dc',
400: '#7248d0',
500: '#592fb7',
600: '#45248f',
700: '#311968',
800: '#1e0f40',
900: '#0c031b',
},
},
fontSize: {
'xs': 11,
'sm': 12,
'md': 13,
'lg': 15,
'xl': 17,
'2xl': 19,
'3xl': 21,
'4xl': 24,
'5xl': 27,
'6xl': 32,
},
};
return (
<ThemeProvider theme={theme}>
...
</ThemeProvider>
)
};